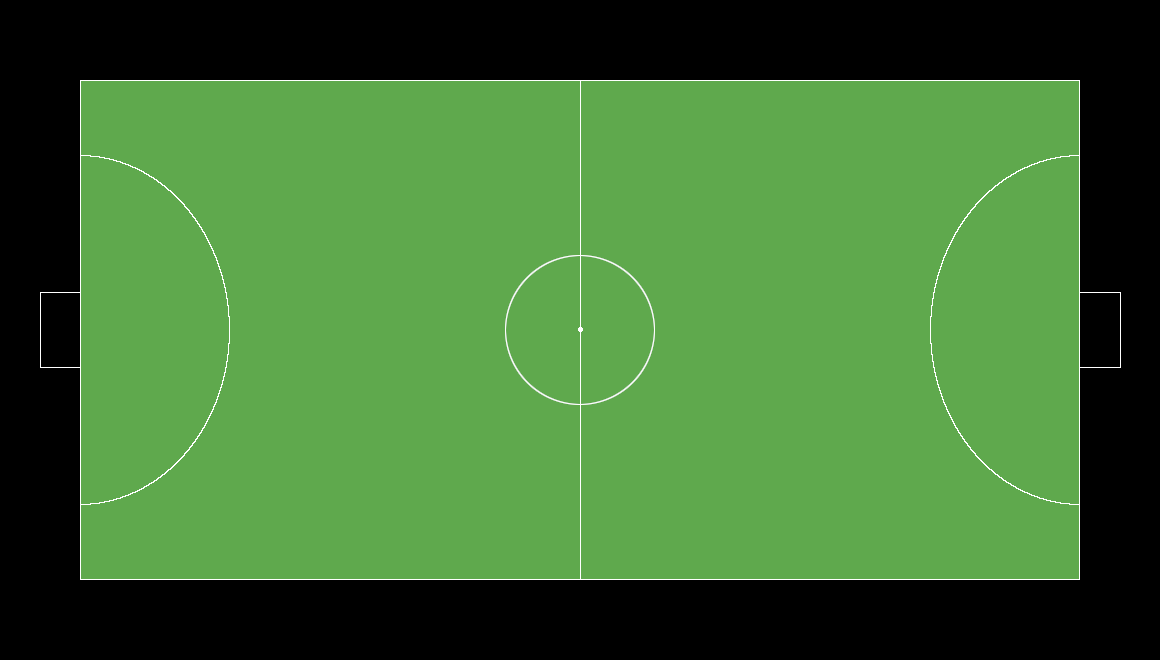
> Game Output
This is a simulation of a soccer game where you have to implement get_command to control the players of your team. The simulation runs for a fixed number of cycles (600 in practice mode, 3000 in compete mode). Each cycle, the engine calls get_command for every player (so 5 calls per cycle).
get_command takes the game state and should return one of the following commands as a string:
- MOVE target_x target_y which moves the player towards (target_x, target_y).
- KICK force target_x target_y to kick the ball. The bigger the force, the less accurate the kick.
- SKIP to keep the player in the same state (do nothing).
Documentation
Showget_command(team, player_number, game_state, user_data) -> command
Takes the game state and returns a command (MOVE, KICK or SKIP) to be sent to the game engine.
Name | Type | Description |
---|---|---|
team | integer | Your team's location (0 for left, 1 for right) |
player_number | integer | The player to get the command for (1 to 5) |
game_state | map of string to dictionary | The current state of the game (see code below for details) |
user_data | map of any to any | Use this map to save any data across cycles. |
The following code snippet shows how to access the game state:
cycle = game_state["cycle"]
ball = game_state["ball"]
ball_x = ball["x"]
ball_y = ball["y"]
ball_v = ball["velocity"]
# your players
my_players = game_state["my_players"]
for player in my_players:
player_number = player["number"] # 1 to 5
player_x = player["x"] # 0 to 1000
player_y = player["y"] # 0 to 500
player_angle = player["angle"] # in degrees clockwise, 0 is right, 90 is bottom, 180 is left, 270 is top
player_v = player["velocity"]
# opponent's players (you can loop over them too)
op_players = game_state["op_players"]
Simulation Details
ShowThe soccer games is simulated based on the following parameters:
Coordinate System
- The game uses a two dimensional coordinate system where the origin is in the top left corner of the field.
- The x axis is horizontal and runs from left to right.
- The y axis is vertical and runs from top to bottom.
Field
- The field size is: 1000 x 500 (length = horizontal x width = vertical).
- The goal size is 75. The left goal's center coordinates are (0, 250). The right goal's center coordinates are (500, 250).
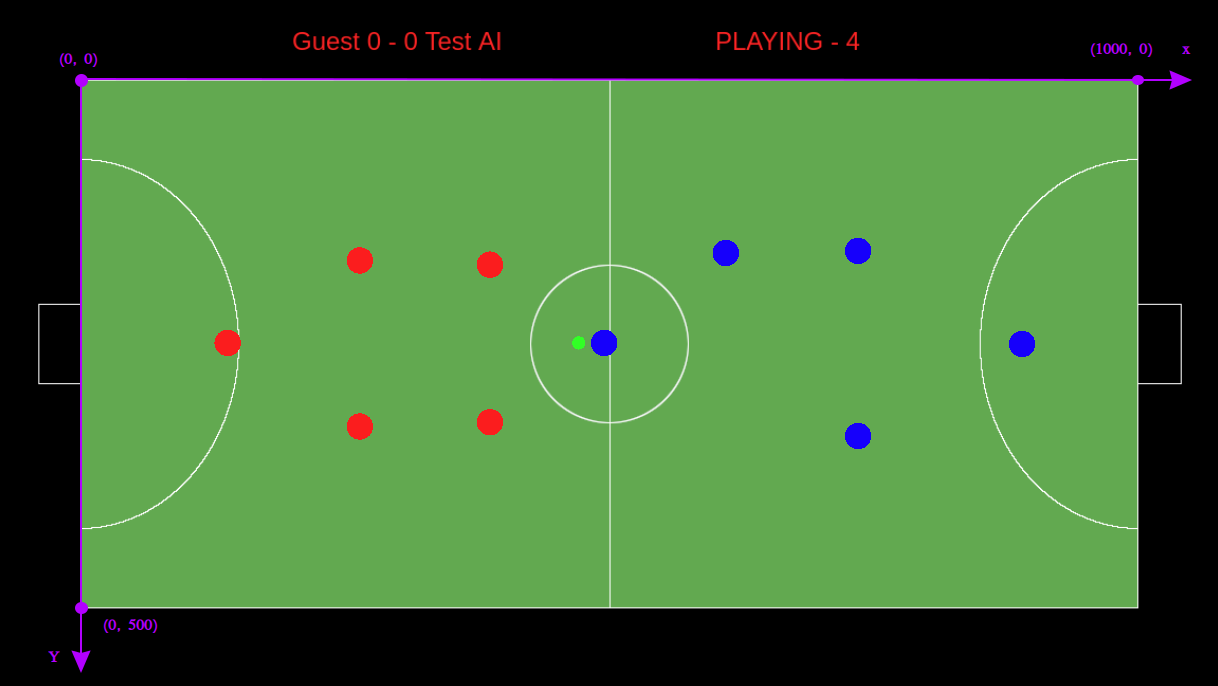
Rules